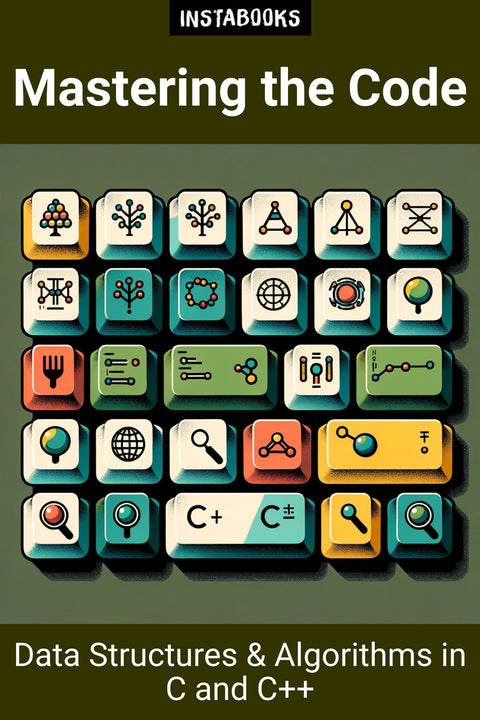
Instabooks AI (AI Author)
Mastering the Code
Data Structures & Algorithms in C and C++
Premium AI Book (PDF/ePub) - 200+ pages
Welcome to Mastering the Code: Data Structures & Algorithms in C and C++
This comprehensive guide is your ultimate resource for mastering data structures and algorithms using the powerful programming languages C and C++. Whether you're a beginner eager to dive into the world of programming or an expert looking to brush up on your skills, this book provides a step-by-step pathway to proficiency.
Why Mastering the Code? Delve deep into the core of programming and unlock the secrets behind efficient data handling and complex algorithms. With detailed explanations, practical examples, and challenging exercises, we guarantee an enriching learning journey.
From understanding basic data structures like linked lists and trees to exploring advanced algorithms for sorting, searching, and graph theory, this book offers a comprehensive exploration of the topics essential for proficiency in C and C++ programming.
Benefit from real-world applications that demonstrate how these concepts are utilized in programming challenges today. You will learn not only the "how" but also the "why", providing you with deeper insights and a solid foundation in data structures and algorithms.
Features Include:
- Clear explanations suitable for beginners.
- Advanced theories for experienced programmers.
- Practical, hands-on coding exercises.
- Real-world applications and examples.
- Comprehensive coverage of essential topics.
Embark on your journey to becoming a proficient programmer with Mastering the Code. This book is your key to unlocking the potential of C and C++, making you adept at handling any programming challenge that comes your way.
Table of Contents
1. Introduction to Data Structures & Algorithms- The Importance of Data Structures
- Understanding Algorithms
- Foundations of C and C++ Programming
2. Basic Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
3. Advanced Data Structures
- Trees and Graphs
- Hash Tables
- Heaps
4. Algorithmic Thinking
- Problem-Solving Strategies
- Divide and Conquer
- Greedy Algorithms
5. Sorting Algorithms
- Understanding Sorting
- Comparison-based Sorting
- Non-comparison Sorting
6. Search Algorithms
- Linear and Binary Search
- Tree Searches
- Graph Searches
7. Graph Algorithms
- Graph Representation
- Graph Traversal
- Shortest Path Algorithms
8. Dynamic Programming
- Introduction to Dynamic Programming
- Memoization and Tabulation
- Dynamic Programming Problems
9. Optimization Techniques
- Time and Space Complexity
- Algorithm Optimization
- Programming Best Practices
10. Real-World Applications
- Data Structure Selection
- Algorithm Design in Practice
- Case Studies
11. Advanced Topics and Future Directions
- Machine Learning Algorithms
- Parallel Programming
- Future Trends in Data Structures and Algorithms
12. Wrapping Up
- Recap and Key Takeaways
- Continuing Your Journey
- Resources for Further Learning
How This Book Was Generated
This book is the result of our advanced AI text generator, meticulously crafted to deliver not just information but meaningful insights. By leveraging our AI book generator, cutting-edge models, and real-time research, we ensure each page reflects the most current and reliable knowledge. Our AI processes vast data with unmatched precision, producing over 200 pages of coherent, authoritative content. This isn’t just a collection of facts—it’s a thoughtfully crafted narrative, shaped by our technology, that engages the mind and resonates with the reader, offering a deep, trustworthy exploration of the subject.
Satisfaction Guaranteed: Try It Risk-Free
We invite you to try it out for yourself, backed by our no-questions-asked money-back guarantee. If you're not completely satisfied, we'll refund your purchase—no strings attached.